Table of Contents
Introduction
In general, functions are the behavior of an object. Now, what are the objects?
Consider You and I as objects. So our behavior or the activities we perform daily like eating, sleeping, coding, etc., are the functions. It’s undeniable that You and I are different, and so will be our functions. Hence, the input and output can be incompatible, but the process is the same.
Hence, A function is a bundle of statements or steps that performs a specific task.
Now, let’s understand the functions in C/C++ programming languages.
What are the functions in C/C++?
Functions (also called ‘procedures’ in some programming languages and ‘methods’ in most object-oriented programming languages) are a set of instructions bundled together to achieve a specific outcome. Functions are an excellent alternative for having repetitive blocks of code in a program. It is possible to reduce the program’s size by calling and using functions at different places in the program.
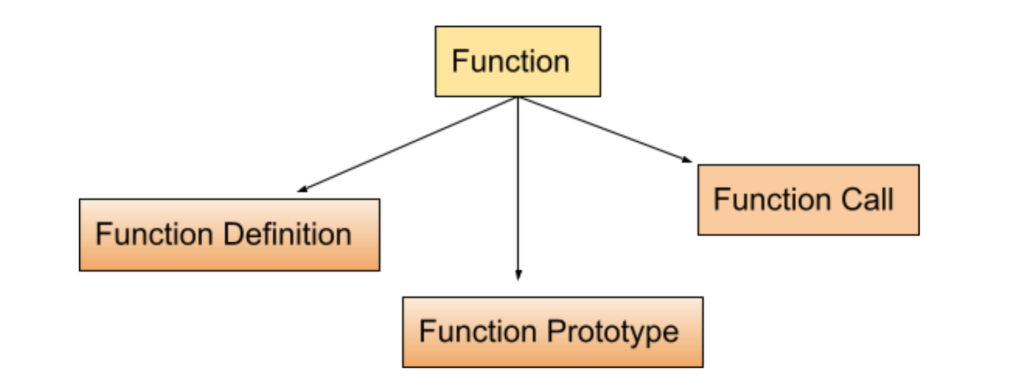
Function Definition
In C/C++, a function must be defined before it is used anywhere in the program. In the function definition, we represent a role by providing the significant steps it has to perform.
type function-name ( parameter list ) { // body of the function }
Here, the type specifies the type of value that the return statement of the function returns. The compiler assumes that the function returns an integer value if no type is specified. The parameter list is a list of variables that are known as the arguments. Let’s see the example to understand better:-
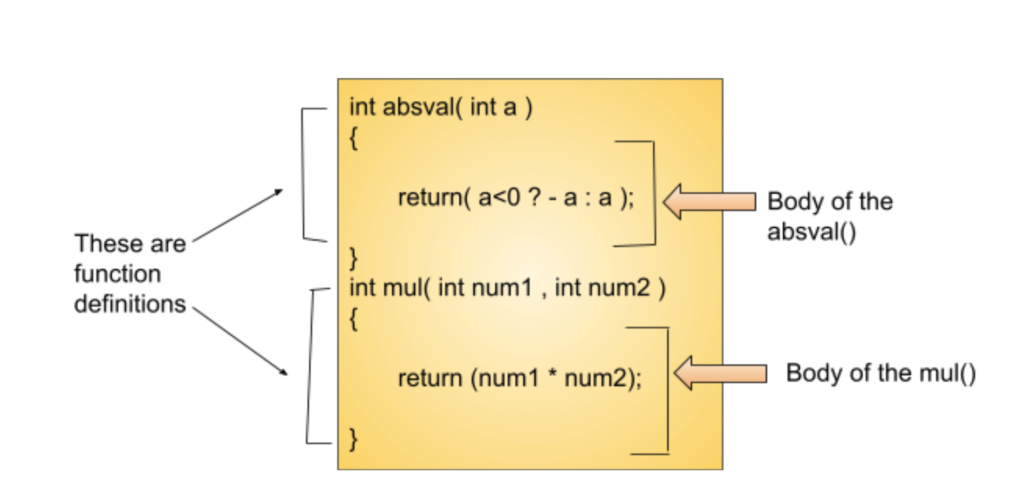
Function Prototype
The function prototype is a function declaration that tells the compiler about the type of the value returned by the function and the types of the arguments before its definition. The prototype looks just like a function definition except that it has no body.
The prototype declaration introduces a function name to the program, whereas a definition does both parts,i.e., What the function is doing and how it is doing.
Need for Prototypes:-
Function prototyping enables a compiler to determine whether the function is adequately invoked carefully, i.e., the number and types of arguments are compared, and any wrong type is reported.
Points to Remember:-
- A function declaration can skip the argument denotation, but a function definition can not.
- If a function doesn’t return a result, declare the return type as void.
void function-name ( parameter list) ;
- If a function takes no arguments, you should specify void in its prototype.
type function-name ( void );
Accessing a Function
A function is called ( or invoked ) by provisioning the function name, followed by the parameters being sent enclosed in parenthesis. For instance, to invoke a function whose prototype looks like
float area( float, float );
the function call statement may look like as shown below :
area ( x , y) ;
where x, y have to be float variables. Whenever a call statement is encountered, the control is transferred to the function, the statement in the function body gets executed. Then the control returns to the statement following the function call.
Let’s see one example for a better understanding of Functions in C/C++:-
Program to print cube of a given number using a function.
C
#include <stdio.h> float cube(float a ) //function definition { return (a*a*a); } int main() { float cube(float); //function prototyping float x, y; printf("Enter a number whose cube is to be calculated: \n"); scanf("%f",&x); y = cube(x); //function calling printf("The cube of %0.2f is %0.2f \n",x,y); return 0; }
Output
Enter a number whose cube is to be calculated: 6 The cube of 6.00 is 216.00
C++
#include <iostream> using namespace std; float cube(float a ) // function definition { return (a*a*a); } int main() { float cube(float); //function prototyping float x, y; cout<<"Enter a number whose cube is to be calculated: \n"; cin>>x; y = cube(x); // function calling cout<<"The cube of "<<x<<" is "<<y<<"\n"; return 0; }
OUTPUT
Enter a number whose cube is to be calculated: 56 The cube of 56 is 175616
Parameters
- The parameters which appear in a function call statement are actual parameters.
- The parameters which appear in the function definition are formal parameters.
Formal parameters are non-identical from the actual parameters. Actual parameters send a copy of them to the formal parameters. However, we can pass the reference of the parameter, which is known as Call by Reference. This is just an overview. We will discuss the invoking types further in this article.
Types of Functions in C/C++
There are broadly two types of functions in C/C++:-
- Built-in (or library) functions:- These functions are part of Standard Library made available by the compiler. For exampleexit( ), sqrt( ), pow( ), strlen( ) etc.
are library functions (or built-in functions).
- User-defined functions:- The user-defined functions are created by the programmer. These functions are created as per their requirements.
THE MAIN FUNCTION
We must have seen the main() function often in the programs. Have you ever wondered about the purpose of that function in C/C++? Let’s understand now!
main() { // main program statements }
In C language, the return type for the main() function is not specified. In C++, the main() returns a value of type int to the operating system. Therefore, C++ explicitly defines main() as matching one of the following prototypes.
int main() ; int main( int argc, char * argv[ ] );
The functions that have a return value should use the return statement for termination. Therefore, main() in C++ is defined as:-
int main() { --------- --------- return 0; }
Many operating systems test the return value ( called exit value) to determine any problem. The standard convention is that an exit value of zero means that the program ran successfully, while a nonzero value means there is a problem in the program.
Need for the main():-
The main() function serves as the beginning point for program execution. It usually controls the program execution by directing the calls to other functions in the program.
When the main calls another function, it passes execution control to the function, so execution begins at the first statement in the function. A function returns control to the main() when a return statement is executed or when the end of the function is reached.
The function can be Invoked in two manners:
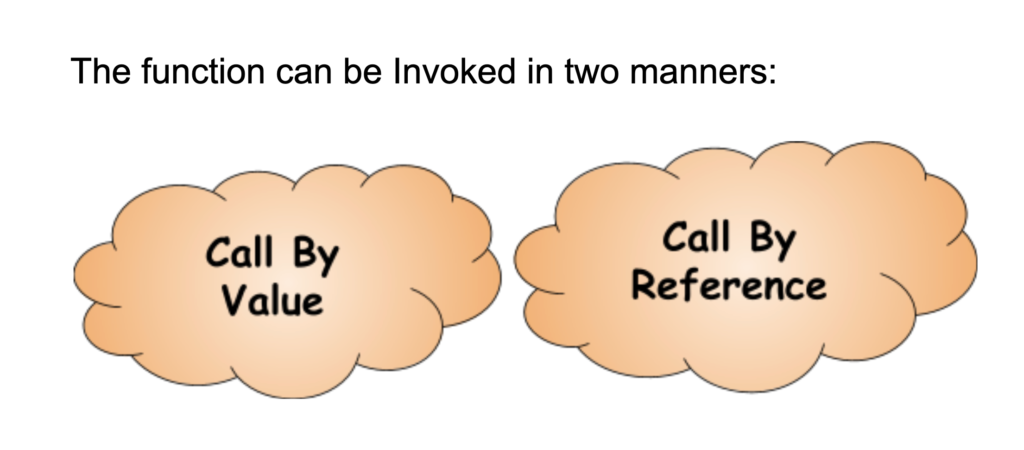
Call By Value:- The call by value method copies the values of actual parameters into the formal parameters; that is, the function creates its copy of argument value and then passes it.
To understand this concept, let us consider one example.
Imagine you have been given a sample of the juice ( formal parameters ) for testing. You tried, and you liked it. Now, the original bottle ( actual parameters ) is still full, so the amount of juice you drank didn’t reflect the original bottle.
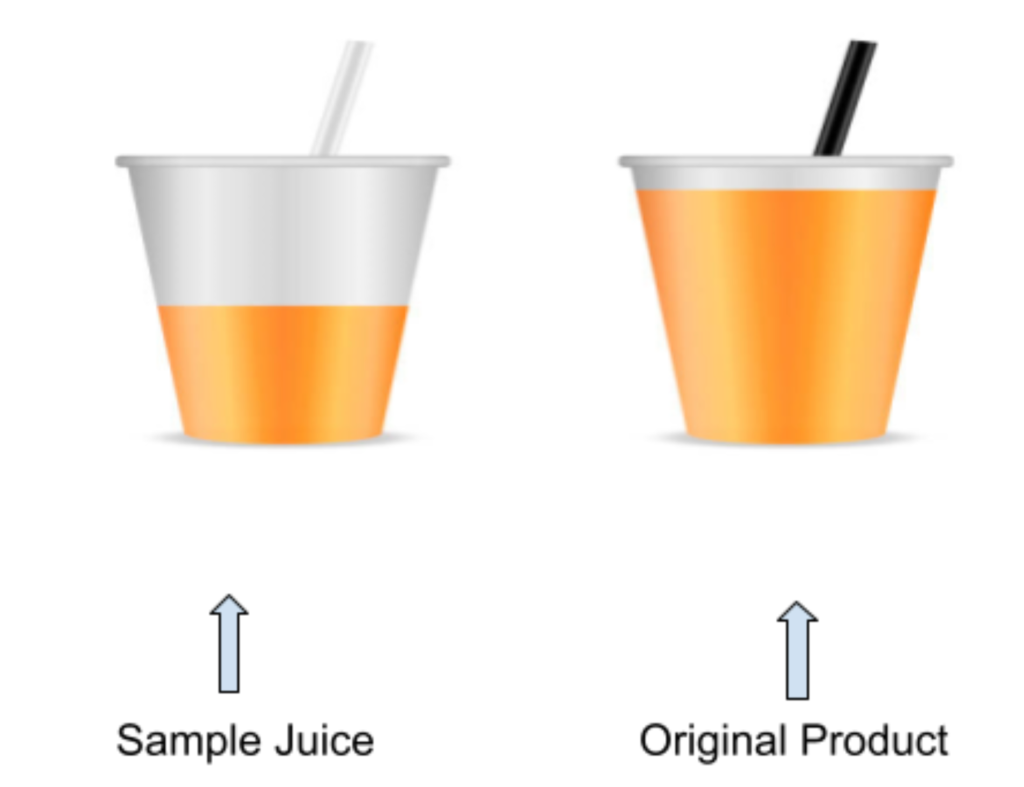
This is precisely the meaning of Call By Value. Hence, during call-by-value, any change in the formal parameter is not reflected in the actual parameters.
Program to swap two numbers using call-by-value.
C
#include <stdio.h> void swap(int value1, int value2){ int temp = value1; value1 = value2; value2 = temp; printf("\nUsing swap() values are a = %d and b = %d",value1,value2); } int main() { void swap(int, int); int a, b; a=6; b=9; printf("\nOriginal values are a = %d and b = %d",a,b); swap(a,b); printf("\nAfter Swapping the values are a = %d and b = %d",a,b); return 0; }
OUTPUT
Original values are a = 6 and b = 9 Using swap() values are a = 9 and b = 6 After Swapping the values are a = 6 and b = 9
C++
#include <iostream> using namespace std; void swap(int value1, int value2){ int temp = value1; value1 = value2; value2 = temp; cout<<"\nUsing swap() values are a = "<<value1<<" and b = "<<value2; } int main() { void swap(int, int); int a, b; a=6; b=9; cout<<"\nOriginal values are a = "<<a<<" and b = "<<b; swap(a,b); cout<<"\nAfter swapping values are a = "<<a<<" and b = "<<b; return 0; }
OUTPUT
Original values are a = 6 and b = 9 Using swap() values are a = 9 and b = 6 After swapping values are a = 6 and b = 9
Call By Reference:- As the name suggests, a reference to the original variable is passed instead of passing a value to the function being called. When a function is called by reference, the formal parameters become references ( or alias ) to the actual parameters. This means that the called function does not create its own copy of original values; instead, it refers to the original values only by different names, i.e., the references. Thus, the called function works with the original data, and any change in the values gets reflected back to the original data.
Let’s quickly see the codes to illustrate the concept:-
Program to swap two numbers using call-by-reference.
C
#include <stdio.h> void swap(int *value1, int *value2){ int temp = *value1; *value1 = *value2; *value2 = temp; printf("Using swap() values are a = %d and b = %d \n",*value1,*value2); } int main() { void swap(int *, int *); int a, b; a=6; b=9; printf("Original values are a = %d and b = %d \n",a,b); swap(&a,&b); printf("After Swapping the values are a = %d and b = %d \n",a,b); return 0; }
OUTPUT
Original values are a = 6 and b = 9 Using swap() values are a = 9 and b = 6 After Swapping the values are a = 9 and b = 6
C++
#include <iostream> using namespace std; void swap(int &value1, int &value2){ int temp = value1; value1 = value2; value2 = temp; cout<<"Using swap() values are a = "<<value1<<" and b = "<<value2<<endl; } int main() { void swap(int &, int &); //Function prototype accepting two reference variables. int a, b; a=6; b=9; cout<<"Original values are a = "<<a<<" and b = "<<b<<endl; swap(a,b); cout<<"After swapping values are a = "<<a<<" and b = "<<b<<endl; return 0; }
OUTPUT
Original values are a = 6 and b = 9 Using swap() values are a = 9 and b = 6 After swapping values are a = 9 and b = 6
The call-by-reference method is helpful in situations where the values of the original variables are to be changed using a function like in the above program.
Frequently Asked Questions
Functions are the subprograms that can be invoked from other parts of the program. The function helps us to reduce the program size and avoid ambiguity.
A formal parameter is a parameter used in the function header of the called function to receive the value from the actual parameter. Actual Parameter is a parameter used in a function call statement to send the value from the calling function to the called function.
The main difference between them is that the copy of the actual parameters is passed to the formal parameters in the call-by-value. Whereas, in call-by-reference, the reference of the actual arguments is passed.
The return statement can be optional only when the function is void or does not return a value.
An inline function is a function that is expanded in line when it is invoked. When a function is declared inline, the compiler replaces the function call with the respective function code.
Key Takeaways
To conclude the discussion on Functions in C/C++, dividing a program into functions is one of the significant objectives of programming. It is possible to reduce the program’s size by invoking and using the functions at different places in the program.
Don’t sit still; practice the functions-based question on codestudio to enlighten the knowledge of learning.
If you found this article obliging, don’t forget to share it with your friends. Any doubt you have regarding any point, you can ask in the comment section.
Level up your coding skills from our top-notch courses.
Leave a Reply