Introduction
This is our last article in the series on, “How to Master in Pattern Problems”.
If you are already familiar with the basic pattern problems and Intermediate level pattern problems, proceed further. If not, please check out the articles on Basic Pattern Problems | Part – 2 and Intermediate Level Pattern Problems | Part – 3 first.
1. Butterfly Pattern
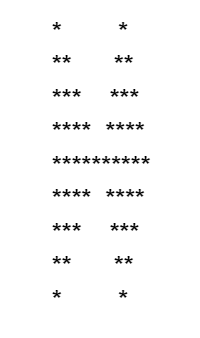
- Firstly, the formula for calculating the matrix is equivalent to 2(n) -1.
- We’ve divided the pattern into two sub-patterns named Upper Half and Lower Half.
- In Upper Half, we are printing the stars first, then spaces, lastly, the stars again. Printing the left and the right-angled triangle is easy; let’s analyse the logic behind the spaces. If we observe, the number of spaces in the first row equals (rows-i) and goes the same with subsequent rows.
- In Lower Half, the outer loop begins with one less, i.e., rows-1, and stops when it reaches 0. Additionally, the spaces are being printed in increasing order. To be precise, here as well, counts are equal to (rows – i), the difference is [i] is being initialized as rows-1, whereas in Upper half from 1 itself.
C
#include <stdio.h>
int main()
{
int rows;
scanf("%d", &rows);
for (int i = 1; i <= rows; i++)
{
for (int j = 0; j < i; j++)
printf("*");
for (int j = 0; j < rows - i; j++)
printf(" ");
for (int j = 0; j < i; j++)
printf("*");
printf("\n");
}
for(int i = rows-1; i > 0; i--){
for(int j = i; j > 0; j--)
printf("*");
for(int j = 1; j <= rows-i; j++)
printf(" ");
for(int j = i; j > 0; j--)
printf("*");
printf("\n");
}
return 0;
}
C++
#include <bits/stdc++.h>
using namespace std;
int main()
{
int rows;
cin >> rows;
for (int i = 1; i <= rows; i++)
{
for (int j = 0; j < i; j++)
cout << "*";
for (int j = 0; j < rows - i; j++)
cout << " ";
for (int j = 0; j < i; j++)
cout << "*";
cout << '\n';
}
for(int i = rows-1; i > 0; i--){
for(int j = i; j > 0; j--)
cout << "*";
for(int j = 1; j <= rows-i; j++)
cout << " ";
for(int j = i; j > 0 ; j--)
cout << "*";
cout << '\n';
}
return 0;
}
import java.util.Scanner;
class pattern{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int rows = sc.nextInt();
for (int i = 1; i <= rows; i++)
{
for (int j = 0; j < i; j++)
System.out.print("*");
for (int j = 0; j < rows - i; j++)
System.out.print(" ");
for (int j = 0; j < i; j++)
System.out.print("*");
System.out.println("");
}
for(int i = rows-1; i > 0; i--){
for(int j = i; j > 0; j--)
System.out.print("*");
for(int j = 1; j <= rows-i; j++)
System.out.print(" ");
for(int j = i; j > 0 ; j--)
System.out.print("*");
System.out.println();
}
}
}
rows = int(input("Enter the number of rows: "))
for i in range(1,rows+1):
print("*" * i, end="")
print(" " * (rows-i),end="")
print("*" * i)
for i in range(rows,0,-1):
print("*" * i, end="")
print(" " * (rows-i),end="")
print("*" * i)
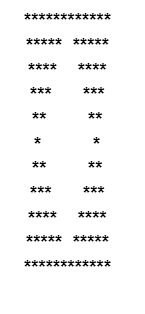
- Similar to the butterfly pattern problem, only the Upper- half and Lower-Half arrangement is swapped.
C
#include <stdio.h>
int main()
{
int rows;
scanf("%d", &rows);
for(int i = 1; i <= rows; i++){
for(int j = rows; j >= i; j--)
printf("*");
for(int j = 1; j < i; j++)
printf(" ");
for(int j = rows; j >= i; j--)
printf("*");
printf("\n");
}
for (int i = 2; i <= rows; i++)
{
for (int j = 0; j < i; j++)
printf("*");
for (int j = 0; j < rows - i; j++)
printf(" ");
for (int j = 0; j < i; j++)
printf("*");
printf("\n");
}
return 0;
}
#include <bits/stdc++.h>
using namespace std;
int main()
{
int rows;
cin >> rows;
for(int i = 1; i <= rows; i++){
for(int j = rows; j >= i; j--)
cout << "*";
for(int j = 1; j < i; j++)
cout << " ";
for(int j = rows; j >= i; j--)
cout << "*";
cout << '\n';
}
for (int i = 2; i <= rows; i++)
{
for (int j = 0; j < i; j++)
cout << "*";
for (int j = 0; j < rows - i; j++)
cout << " ";
for (int j = 0; j < i; j++)
cout << "*";
cout << '\n';
}
return 0;
}
import java.util.Scanner;
class pattern{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int rows = sc.nextInt();
for(int i = 1; i <= rows; i++){
for(int j = rows; j >= i; j--)
System.out.print("*");
for(int j = 1; j < i; j++)
System.out.print(" ");
for(int j = rows; j >= i; j--)
System.out.print("*");
System.out.println("");
}
for (int i = 2; i <= rows; i++)
{
for (int j = 0; j < i; j++)
System.out.print("*");
for (int j = 0; j < rows - i; j++)
System.out.print(" ");
for (int j = 0; j < i; j++)
System.out.print("*");
System.out.println("");
}
}
}
Python
rows = int(input("Enter the number of rows :"))
#upper half
for i in range (1,rows+1):
print("*" * i, end="")
print(" " * (rows-i)*2, end="")
print("*" * i)
#lower half
for i in range (rows,0,-1):
print("*" * i, end="")
print(" " * (rows-i)*2, end="")
print("*" * i)
3. Diagonal & Sides of a Rhombus/Diamond
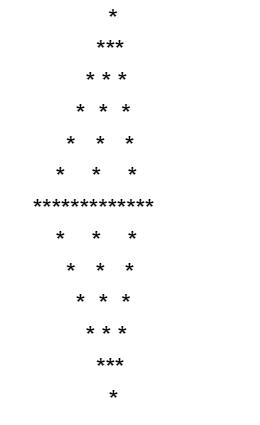
- The pictorial representation of the above pattern in general for any case. That is how one can build the logic for the problem to get the desired output.
- If all the above conditions fail, then in the else block, spaces will be printed.
- Before moving to the solution, it is highly recommended to solve the problem by yourself. Additionally, you can try the Hollow Diamond Pattern Problem.
C
#include <stdio.h>
int main()
{
int i, j, rows;
scanf("%d", &rows); // ‘rows’ must be odd
int num1 = rows / 2 * 3;
for(i = 0; i < rows; i++)
{
for(j = 0; j < rows; j++)
{
// center horizontal, center vertical, upper left diagonal, bottom left diagonal, upper right diagonal, bottom right diagonal
if(i == rows / 2 || j == rows / 2 || i + j == rows / 2 || i - j == rows / 2 || j - i == rows / 2 || i + j == num1)
printf("*");
else
printf(" ");
}
printf("\n");
}
return 0;
}
C++
#include <iostream>
using namespace std;
int main()
{
int i, j, rows;
cin >> rows; // ‘rows’ must be odd
int num1 = rows / 2 * 3;
for(i = 0; i < rows; i++)
{
for(j = 0; j < rows; j++)
{
// center horizontal, center vertical, upper left diagonal, bottom left diagonal, upper right diagonal, bottom right diagonal
if(i == rows / 2 || j == rows / 2 || i + j == rows / 2 || i - j == rows / 2 || j - i == rows / 2 || i + j == num1)
cout << "*";
else
cout << " ";
}
cout << "\n";
}
return 0;
}
Java
import java.util.Scanner;
public class Main
{
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
int i, j;
int rows = sc.nextInt(); // ‘rows’ must be odd
int num1 = rows / 2 * 3;
for(i = 0; i < rows; i++)
{
for(j = 0; j < rows; j++)
{
// center horizontal, center vertical, upper left diagonal, bottom left diagonal, upper right diagonal, bottom right diagonal
if(i == rows / 2 || j == rows / 2 || i + j == rows / 2 || i - j == rows / 2 || j - i == rows / 2 || i + j == num1)
System.out.print("*");
else
System.out.print(" ");
}
System.out.println();
}
}
}
Python
for row in range(0,7):
for col in range(0,7):
if(row+col==3) or (col-row==3) or (row+col==9) or(row-col==3) or (row==3) or (col==3):
print("*", end="")
else:
print(" ", end="")
print()
4. Heart
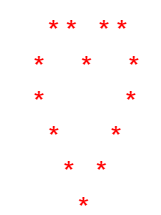
- For more clarification, draw the heart in your notebook and start analyzing the logic. This way, you’ll be able to solve similar pattern problems. The goal is not to mug up the logic or approaches but to understand what is exactly happening. Moreover, What can be the approaches one could make?
C
#include <stdio.h>
int main() {
for(int i=0;i<6;i++){
for(int j=0;j<7;j++){
if((i==0 && j%3!=0) || (i==1 && j%3==0) || (i-j==2) || (i+j==8)){
printf("* ");
}
else{
printf(" ");
}
}
printf("\n");
}
return 0;
}
C++
#include <bits/stdc++.h>
using namespace std;
int main() {
for(int i=0;i<6;i++){
for(int j=0;j<7;j++){
if((i==0 && j%3!=0) || (i==1 && j%3==0) || (i-j==2) || (i+j==8)){
cout<<"*"<<" ";
}
else{
cout<<" "<<" ";
}
}
cout<<endl;
}
return 0;
}
Java
public class PrintHeart {
public static void main(String[] args) {
for(int i=0;i<6;i++){
for(int j=0;j<7;j++){
if((i==0 && j%3!=0) || (i==1 && j%3==0) || (i-j==2) || (i+j==8)){
System.out.print("*"+" ");
}
else{
System.out.print(" ");
}
}
System.out.println();
}
}
}
Python
for row in range(6):
for col in range(7):
if(row==0 and col%3!=0) or (row==1 and col%3==0) or (row-col==2) or (row+col==8):
print("*", end=" ")
else:
print(" ",end=" ")
print()
Key Takeaways
Tadaa, you made it here; kudos for your efforts now; keep practicing these questions. To Master Pattern Problems, you need to practice a lot. While practice might not necessarily make your skills perfect, it is still an essential piece of learning.
By balancing methods that include mental rehearsal, hands-on training, exploration, and different forms of knowledge, you can optimise skill development and become a Master in Pattern Problems. If you found this article helpful, do share it with your friends.
Don’t stop here Ninja, do check our top-notch courses. And make the journey of coding fruitful.
By Alisha Chhabra
Leave a Reply