Table of Contents
Introduction
Recursion is a topic most beginner programmers fear and when you get the hang of it, it works like magic (Congrats! Not a noob anymore). When someone introduces recursion, they say it’s basically a function that calls back itself, and your brain goes like WHAAAAAT??
THE GOOD
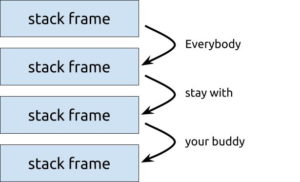
3. Three magic words: tail call optimization: Some functional languages implement this wizardry. So basically, what happens is, if a function’s return expression is a result of a function call, the stack frame is reused instead of pushing a new stack frame in the call stack. Sadly, only a few imperative languages have this wizardry.
The secret magic of tail call
THE BAD
A lot of programmers avoid using recursion and believe that it is less efficient than its iterative counterparts.
- space is carved out on the stack for function arguments and variables.
- arguments are copied into this new space
- control jumps to the function
- function code runs
- function results are copied into the new value
- stack rebounds to its previous position
- control jumps back to the caller
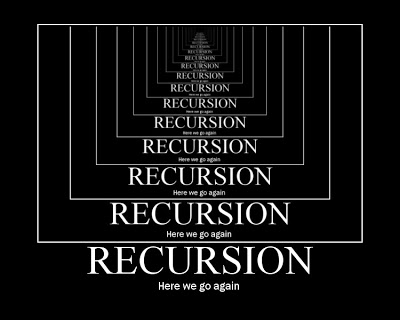
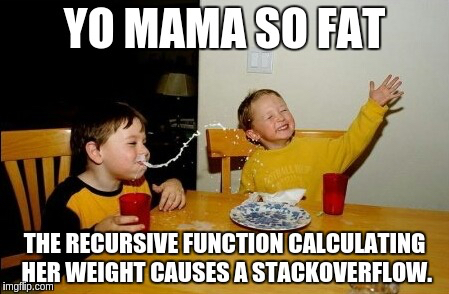
Frequently Asked Questions
To see code in recursion, you can look up recursive functions in c, recursive functions in python, recursive functions in java, recursive functions in c++ or recursive functions in data structures in general.
Recursion is the phenomenon in programming where a function, called the recursive function calls itself directly or indirectly based on certain conditions.
Example:
void recursion(int n){
if(n==0) return;
else
recursion(n-1);
}
Recursion in language is the phenomenon of repeating things in a way that it seems similar to the parent thing.
Recursion is used for breaking down a complex problem into a simpler problem.
Recursive thinking is the process of analysing a problem and breaking it down into smaller problems.
Recursion syntax is the syntax of writing a recursive call which consists of the name of the recursive call and arguments if any.
Also Read about Learning Recursion in C++.
Thanks very interesting blog!
Thank you 🙂
Yes!
Thanks
Remarkable! Its really awesome article, I have got much clear idea about from this article.
Thank you for reading!
It’s nearly impossible to find knowledgeable people for this subject, but you seem like you know
what you’re talking about! Thanks
I enjoy, cause I found just what I was looking for.
You’ve ended my 4 day long hunt! God Bless you
man. Have a great day. Bye
Some truly prime content on this website , saved to my bookmarks .